Date
The Date type is available starting from version 4.9.0.
If time is irrelevant to your scenario, instead of Date and time, you can select Date type.
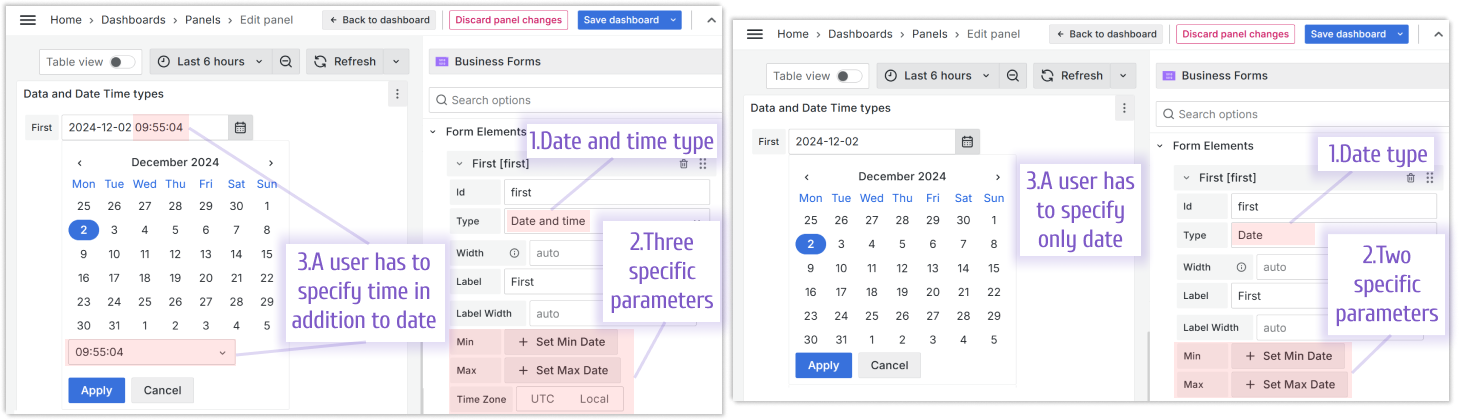
Change Elements Model​
Use this model to set element(s) if you use context.panel.onChangeElements([])
method.
Dynamic element creation and manipulation through the panel uses the concept that the model of the element(s) will be fetched in its entirety and set through a method. The elements will not be saved in the panel options.
{
uid:'',
id: '',
title: '',
type: 'date',
min: '',
max: '',
labelWidth: 10,
width: 30,
tooltip: '',
section: '',
unit: '',
value: '',
disabled: false,
background: '',
labelBackground:'',
labelColor: '',
helpers: {
showIf: () => true,
disableIf: () => false,
getOptions: () => [],
}
}
Code example​
/**
* Hardcoded element
*/
const element = {
uid: "uid-123",
id: "element-123",
title: "Element",
type: "date",
min: "",
max: "",
labelWidth: 15,
width: 150,
tooltip: "",
section: "",
unit: "",
value: "",
background: "",
labelBackground: "",
labelColor: "",
helpers: {
showIf: () => true,
disableIf: () => false,
getOptions: () => [],
},
};
const elementsForUI = [];
elementsForUI.push(element);
context.panel.onChangeElements(elementsForUI);
Code Example with Query action for Initial Request​
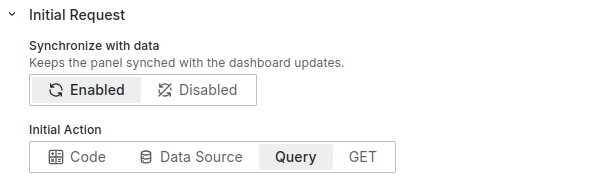
/**
* Convert JSON to form elements array
*/
const formElements = JSON.parse(
context.panel.data.series[0].fields[0].values[0]
);
/**
* Set elements with helpers
*/
context.panel.onChangeElements(
formElements.map((element) => {
const elementInForm = context.panel.elements.find(
(item) => item.uid === element.uid
);
let value = element.value;
if (element.uid === "comment" && elementInForm) {
value = elementInForm.value;
}
return {
...element,
value,
helpers: {
showIf: () => true,
disableIf: () => false,
getOptions: () => element.options,
},
};
})
);
Element Parameters​
-
value
​string. Optional.
Current element value. Format: '2025-01-28T08:07:37.359Z' -
type
​string. Required.
Element type: 'date'. -
min
​string. Optional.
Min available date. Format: '2025-01-28T08:07:37.359Z' -
max
​string. Optional.
Max available date. Format: '2025-01-28T08:07:37.359Z'
-
uid
​string. Required.
Unique identifier is used for correct mapping on UI. -
id
​string. Required.
Unique identifier of element. -
title
​string. Required.
Title/Label of element. Equivalent to the 'Label' in the UI editor.
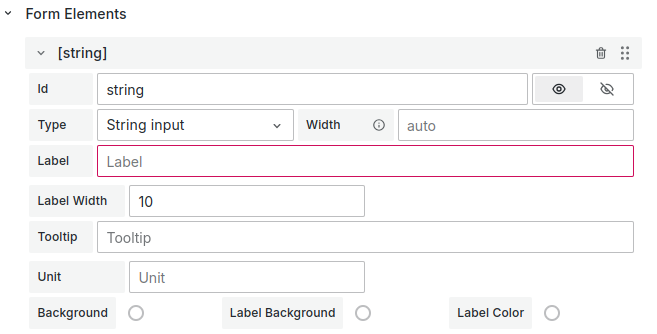
-
labelWidth
​Number | null . Required.
Element label width. -
width
​Number | null. Required.
Element width. -
tooltip
​string. Required.
Element tooltip. Leave empty string ('') if not displayed -
section
​string. Required.
Unique section identifier (section ID). Specified if the element is placed in a section. Leave empty string ('') if the element has no section. -
unit
​string. Required.
Units of measurement. Located to the right of the element. Leave empty string ('') if has no unit.

-
background
​string. Optional.
Hex color code. Background color. -
labelBackground
​string. Optional. Hex color code. Label Background color.
-
labelColor
​string. Optional.
Hex color code. Label color. -
helpers
​Helpers - provide information about the item display or its disable state, returns options
{
showIf: () => true,
disableIf: () => false,
getOptions: () => options || [],
}
-
helpers.showIf
​Function. Required. Should return true or false. Responsible for displaying the element on the UI.
-
helpers.disableIf
​Function. Required. Should return true or false. Responsible for disable state the element on the UI.
-
helpers.getOptions
​Function. Required. Should return array of options.
Each option contain following property
-
value
.
string. Required. Option value -
label
.
string. Required. Option label
-
The Date element does not support options for selection. However, they must be specified in the element object
Change Options Model​
Use this model to set element(s) if you use context.panel.onOptionsChange({})
method.
The element added through this method will be added to the panel options. Operation of the element may not be the same as the expected behavior.
If you use it in the initial request, don't forget to disable the Synchronize option.
Enabling the Synchronize option and using it together with context.panel.onOptionsChange()
in the Initial Request will cause the panel to reload constantly.
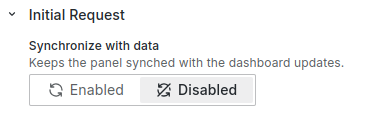
{
uid:'',
id: '',
title: '',
type: 'date',
min: '',
max: '',
labelWidth: 10,
width: 35,
tooltip: '',
section: '',
unit: '',
value: '',
showIf: '',
disableIf: '',
fieldName: '',
queryField: {},
background: '',
labelBackground:'',
labelColor: '',
}
Code example​
context.panel.onOptionsChange({
...context.panel.options,
elements: context.panel.options.elements.map((element) => {
return element.id === "name" ? { ...element, value: "test" } : element;
}),
});
const formElements = JSON.parse(
context.panel.data.series[0].fields[0].values[0]
);
context.panel.onOptionsChange({
...context.panel.options,
elements: formElements,
});
-
value
​string. Optional.
Current element value. Format: '2025-01-28T08:07:37.359Z' -
type
​string. Required.
Element type: 'date'. -
min
​string. Optional.
Min available date. Format: '2025-01-28T08:07:37.359Z' -
max
​string. Optional.
Max available date. Format: '2025-01-28T08:07:37.359Z'
-
uid
​string. Required.
Unique identifier is used for correct mapping on UI. -
id
​string. Required.
Unique identifier of element. -
title
​string. Required.
Title/Label of element. Equivalent to the 'Label' in the UI editor.Label field -
labelWidth
​Number | null . Required.
Element label width. -
width
​Number | null. Required.
Element width. -
tooltip
​string. Required.
Element tooltip. Leave empty string ('') if not displayed -
section
​string. Required.
Unique section identifier (section ID). Specified if the element is placed in a section. Leave empty string ('') if the element has no section. -
unit
​string. Required.
Units of measurement. Located to the right of the element. Leave empty string ('') if has no unit.Unit of measurement -
background
​string. Optional.
Hex color code. Background color. -
labelBackground
​string. Optional.
Hex color code. Label Background color. -
labelColor
​string. Optional.
Hex color code. Label color. -
showIf
​string. Optional.
"Show if" function of the element returns the value true or false. Responsible for displaying the element on the UI.{
showIf: "if (condition) {\n return true\n}\n return false";
} -
disableIf
​string. Optional.
"Disable if" function of the element returns the value true or false. Responsible for disable state the element on the UI.{
disableIf: "if (condition) {\n return true\n}\n return false";
} -
fieldName
​string. Optional.
Name field from DataSource initial request. Use for initial value. Rec. usevalue
instead it. -
queryField
​object. Optional.
Name query from Query initial request. Use for initial value. Rec. usevalue
instead it.Parameters:
-
refId
string. Frame refId -
value
string. Field name -
label
string. Format:refId:value
(A:time
)
-